반응형
문제 설명 2. Add Two Numbers
You are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order, and each of their nodes contains a single digit. Add the two numbers and return the sum as a linked list.
You may assume the two numbers do not contain any leading zero, except the number 0 itself.
Example 1:
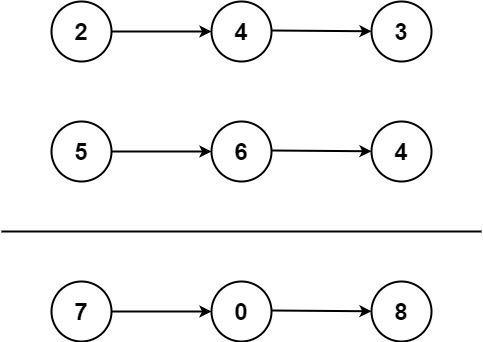
Input: l1 = [2,4,3], l2 = [5,6,4]
Output: [7,0,8]
Explanation: 342 + 465 = 807.
Example 2:
Input: l1 = [0], l2 = [0]
Output: [0]
Example 3:
Input: l1 = [9,9,9,9,9,9,9], l2 = [9,9,9,9]
Output: [8,9,9,9,0,0,0,1]
Constraints:
- The number of nodes in each linked list is in the range
[1, 100]
. 0 <= Node.val <= 9
- It is guaranteed that the list represents a number that does not have leading zeros.
문제 풀이
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
class Solution:
def addTwoNumbers(self, l1:ListNode, l2:ListNode)->ListNode:
root = current = ListNode()
carry = 0
val = 0
while l1 or l2 or carry:
if not l1:
l1 = ListNode()
if not l2:
l2 = ListNode()
carry, val = divmod(l1.val + l2.val + carry, 10)
l1 = l1.next
l2 = l2.next
current.next = ListNode(val)
current = current.next
return root.next
반응형
'스터디 공간' 카테고리의 다른 글
[Python-Leetcode] 13. Roman to Integer (difficulty : Easy - ☆) (0) | 2022.05.05 |
---|---|
[Python-Leetcode] 1. Two Sum (difficulty : Easy - ☆) (0) | 2022.05.01 |
[Python-Leetcode] 9. Palindrome Number (difficulty : Easy - ☆) (0) | 2022.04.27 |
[Python-Leetcode] 4. Median of Two Sorted Arrays (difficulty : Hard - ☆☆☆) (0) | 2022.04.27 |
[sklearn] Data Scaling (Standard / minmax / maxabs / Robust) (0) | 2022.02.27 |
댓글